Separate Laravel6 user and administrator authentication
Aug 24, 2020
PHP
Beginner
Laravel
For Beginners
Laravel6
Purpose
- Summarize the method of separating authentication between user and administrator.
Implementation environment
- Hardware environment
Item | Information |
---|---|
OS | macOS Catalina(10.15.5) |
PC | MacBook Pro (13-inch, 2020, Four Thunderbolt 3 ports) |
Processor | 2 GHz Quad Core Intel Core i5 |
Memory | 32 GB 3733 MHz LPDDR4 |
Graphics | Intel Iris Plus Graphics 1536 MB |
- Software environment
Item | Information | Remarks |
---|---|---|
PHP version | 7.4.3 | Introduced using Homwbrew |
Laravel version | 6.18.35 | Introduced by this method using commposer → Construct environment of Mac Laravel |
MySQL version | 8.0.19 for osx10.13 on x86_64 | Introduced by this method using Homwbrew → Install MySQL with Mac Homebrew |
#Prerequisites
- An environment close to the previous implementation environment is in place.
- Laravel is built for the latest version, but create it by specifying 5.6 when executing the application creation command.
#Prerequisite information
- Create Laravel application directly on Mac local environment without using Docker or AWS.
- Since the work is a little difficult, describe it carefully so that it is easy to understand.
- If the contents of this article are implemented from the beginning, anyone can authenticate separately for the user and the administrator.
- Administrator authentication information is the administrator name and password.
- To avoid complicating the work, do not allow the password for the administrator to be changed from the application side.
- Add and edit existing Auth authentication controller to create administrator authentication function.
- Extract and implement only the necessary name part referring to the following document. Also, the order of implementation will be changed slightly to make the explanation more understandable. -How to use multiple authentication guards in LARAVEL app
- It will be implemented by applying the contents of the following article, but from the part of application creation it will be described in this article based on the ease of understanding.
After reading
- With Laravel6, you can create an application with user authentication and administrator authentication function, and the administrator authentication information is the administrator name and password.
Overview
- Creating a database
- Laravel application creation and initial settings
- Creation of user authentication function
- Preparation of administrator information table
- Adding guards and providers
- Modify the controller
- Creating an authentication page
- Creating post-authentication transition page
- Description of routing and setting of transition page after authentication and description of processing at the time of exception
- Confirm
#Details
-
Creating a database
-
Execute the following command to log in to MySQL from the terminal. (If you have forgotten the MySQL root user password, click here → How to reset MySQL 8.x root password for Mac local environment)
$ mysql -u root -p
-
Execute the following SQL to create “multi_auth” database.
create database multi_auth_info_limited_laravel_6;
-
Execute the following SQL to output the database list and confirm that “multi_auth_info_limited_laravel_6” is included. After confirmation, log out of MySQL.
show databases;
-
-
Laravel application creation and initial settings
-
Change to the directory where you want to create the Laravel application.
-
Execute the following command to create a Laravel 5.6 application called “multi_auth_info_limited_laravel_6”. (It may take some time to complete, so wait a while.)
$ composer create-project laravel/laravel multi_auth_info_limited_laravel_6 "5.6.*"
-
Move to the application name directory created by executing the following command. Unless otherwise specified, the following commands are executed inside this multi_auth directory.
$ cd multi_auth_info_limited_laravel_6
-
Execute the following command to open the .env file.
$ vi .env
-
Modify the database description in the .env file as shown below.
DB_DATABASE=multi_auth_info_limited_laravel_6 DB_USERNAME=root DB_PASSWORD=mysql -u root -p password entered when executing the command
-
Describe the entire contents of the modified .env file.
APP_NAME=Laravel APP_ENV=local APP_KEY=The description of the app key differs for each individual. APP_DEBUG=true APP_URL=http://localhost LOG_CHANNEL=stack DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=multi_auth_info_limited_laravel_6 DB_USERNAME=root DB_PASSWORD=Mysql root user password in your environment BROADCAST_DRIVER=log CACHE_DRIVER=file QUEUE_CONNECTION=sync SESSION_DRIVER=file SESSION_LIFETIME=120 REDIS_HOST=127.0.0.1 REDIS_PASSWORD=null REDIS_PORT=6379 MAIL_MAILER=smtp MAIL_HOST=smtp.mailtrap.io MAIL_PORT=2525 MAIL_USERNAME=null MAIL_PASSWORD=null MAIL_ENCRYPTION=null MAIL_FROM_ADDRESS=null MAIL_FROM_NAME="${APP_NAME}" AWS_ACCESS_KEY_ID= AWS_SECRET_ACCESS_KEY= AWS_DEFAULT_REGION=us-east-1 AWS_BUCKET= PUSHER_APP_ID= PUSHER_APP_KEY= PUSHER_APP_SECRET= PUSHER_APP_CLUSTER=mt1 MIX_PUSHER_APP_KEY="${PUSHER_APP_KEY}" MIX_PUSHER_APP_CLUSTER="${PUSHER_APP_CLUSTER}"
-
Execute the following command to migrate the initial migration file.
$ php artisan migrate
-
Execute the following command to start the local server.
$ php artisan serve
-
Access the following and confirm that the initial screen of Laravel is displayed. -http://localhost:8000/
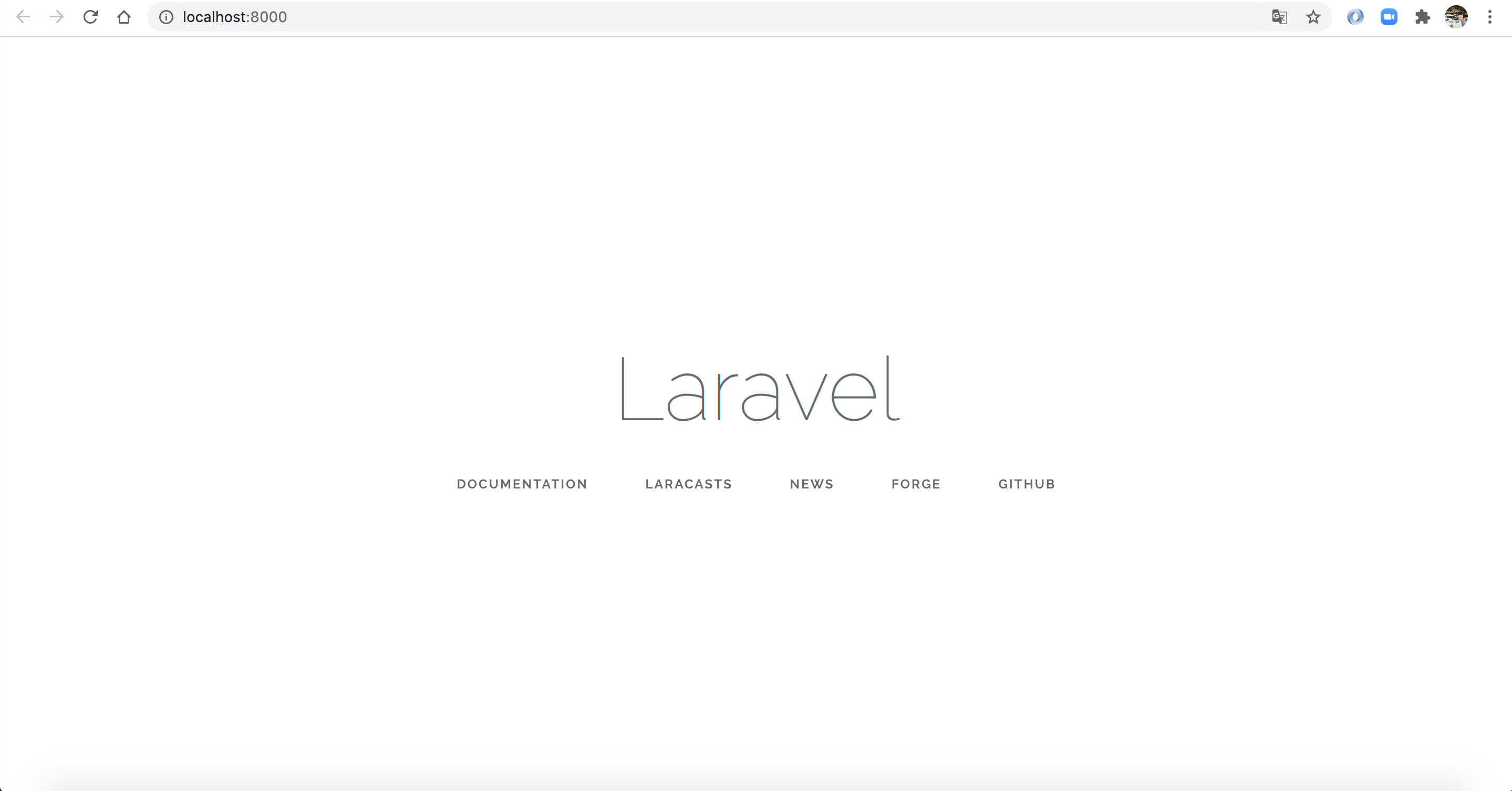
-
-
Creation of user authentication function
-
Execute the following command to create a user authentication function.
$ composer require laravel/ui "^1.0" --dev $ php artisan ui vue --auth $ npm install && npm run dev
-
Execute the following command to start the local server.
$ php artisan serve
-
Open the Laravel initial screen by accessing the following. -http://localhost:8000/
-
Click “REGISTER” on the upper right.
-
Enter various information and click “Register”.
-
Confirm that the screen moves to the corner after clicking “Register”.
-
-
Preparation of administrator information table
-
Execute the following command to create Admin model file and admins table migration file.
$ php artisan make:model Admin -m
-
Execute the following command to open the migration file created earlier. (The YYYY_MM_DD_XXXXXX part depends on the migration file creation date.)
$ vi database/migrations/YYYY_MM_DD_XXXXXX_create_admins_table.php
-
Add the opened migration file as follows.
<?php use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class CreateAdminsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('admins', function (Blueprint $table) { $table->increments('id'); // add from below $table->string('name'); $table->string('password'); $table->boolean('is_super')->default(false); $table->rememberToken(); // Add the above $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('admins'); } }
-
Execute the following command to migrate the migration file just described.
$ php artisan migrate
-
Execute the following command to open the model file created earlier.
$ vi app/Admin.php
-
Delete all the contents of the opened model file and copy and paste the contents below.
<?php namespace App; use Illuminate\Notifications\Notifiable; use Illuminate\Foundation\Auth\User as Authenticatable; class Admin extends Authenticatable { use Notifiable; protected $guard ='admin'; protected $fillable = [ 'name','password', ]; protected $hidden = [ 'password','remember_token', ]; }
-
-
Adding guards and providers
-
Execute the following command to open the file that defines the guard and provider.
$ vi config/auth.php
-
Add the description of the guard as follows.
/* |------------------------------------------------- ------------------------- | Authentication Guards |------------------------------------------------- ------------------------- | | Next, you may define every authentication guard for your application. | Of course, a great default configuration has been defined for you | here which uses session storage and the Eloquent user provider. | | All authentication drivers have a user provider. This defines how the | users are actually retrieved out of your database or other storage | mechanisms used by this application to persist your user's data. | | Supported: "session", "token" | */ 'guards' => [ 'web' => [ 'driver' =>'session', 'provider' =>'users', ], 'api' => [ 'driver' =>'token', 'provider' =>'users', ], // Add the following 'admin' => [ 'driver' =>'session', 'provider' =>'admins', ], // Add up to the above ],
-
Add the description of the provider in the same file.
/* |------------------------------------------------- ------------------------- | User Providers |------------------------------------------------- ------------------------- || All authentication drivers have a user provider. This defines how the | users are actually retrieved out of your database or other storage | mechanisms used by this application to persist your user's data. | | If you have multiple user tables or models you may configure multiple | sources which represent each model / table. These sources may then | be assigned to any extra authentication guards you have defined. | | Supported: "database", "eloquent" | */ 'providers' => [ 'users' => [ 'driver' => 'eloquent', 'model' => App\User::class, ], //下記を追記する 'admins' => [ 'driver' => 'eloquent', 'model' => App\Admin::class, ], //上記までを追記する // 'users' => [ // 'driver' => 'database', // 'table' => 'users', // ], ],
-
追記後の
multi_auth_info_limited_laravel_6/config/auth.php
のファイルの全体を下記に記載する。<?php return [ /* |-------------------------------------------------------------------------- | Authentication Defaults |-------------------------------------------------------------------------- | | This option controls the default authentication "guard" and password | reset options for your application. You may change these defaults | as required, but they're a perfect start for most applications. | */ 'defaults' => [ 'guard' => 'web', 'passwords' => 'users', ], /* |-------------------------------------------------------------------------- | Authentication Guards |-------------------------------------------------------------------------- | | Next, you may define every authentication guard for your application. | Of course, a great default configuration has been defined for you | here which uses session storage and the Eloquent user provider. | | All authentication drivers have a user provider. This defines how the | users are actually retrieved out of your database or other storage | mechanisms used by this application to persist your user's data. | | Supported: "session", "token" | */ 'guards' => [ 'web' => [ 'driver' => 'session', 'provider' => 'users', ], 'api' => [ 'driver' => 'token', 'provider' => 'users', ], //下記を追記する 'admin' => [ 'driver' => 'session', 'provider' => 'admins', ], //上記までを追記する ], /* |-------------------------------------------------------------------------- | User Providers |-------------------------------------------------------------------------- | | All authentication drivers have a user provider. This defines how the | users are actually retrieved out of your database or other storage | mechanisms used by this application to persist your user's data. | | If you have multiple user tables or models you may configure multiple | sources which represent each model / table. These sources may then | be assigned to any extra authentication guards you have defined. | | Supported: "database", "eloquent" | */ 'providers' => [ 'users' => [ 'driver' => 'eloquent', 'model' => App\User::class, ], //下記を追記する 'admins' => [ 'driver' => 'eloquent', 'model' => App\Admin::class, ], //上記までを追記する // 'users' => [ // 'driver' => 'database', // 'table' => 'users', // ], ], /* |-------------------------------------------------------------------------- | Resetting Passwords |-------------------------------------------------------------------------- | | You may specify multiple password reset configurations if you have more | than one user table or model in the application and you want to have| separate password reset settings based on the specific user types. | | The expire time is the number of minutes that the reset token should be | considered valid. This security feature keeps tokens short-lived so | they have less time to be guessed.You may change this as needed. | */ 'passwords' => [ 'users' => [ 'provider' =>'users', 'table' =>'password_resets', 'expire' => 60, ], ], ];
-
-
Modify the controller
-
Modify the following two controllers. -
multi_auth_info_limited_laravel_6/app/Http/Controllers/Auth/LoginController.php
-multi_auth_info_limited_laravel_6/app/Http/Controllers/Auth/RegisterController.php
-
Execute the following command to open the controller file that controls login.
$ vi app/Http/Controllers/Auth/LoginController.php
-
Modify the opened controller file as follows.
<?php namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use Illuminate\Foundation\Auth\AuthenticatesUsers; // add the following use Illuminate\Http\Request; use Auth; // add up to the above class LoginController extends Controller { /* |------------------------------------------------- ------------------------- | Login Controller |------------------------------------------------- ------------------------- | | This controller handles authenticating users for the application and | redirecting them to your home screen.The controller uses a trait | to conveniently provide its functionality to your applications. | */ use AuthenticatesUsers; /** * Where to redirect users after login. * * @var string */ protected $redirectTo ='/home'; /** * Create a new controller instance. * * @return void */ public function __construct() { $this->middleware('guest')->except('logout'); // Add the following $this->middleware('guest:admin')->except('logout'); } // add the following public function showAdminLoginForm() { return view('auth.login', ['url' =>'admin']); } public function adminLogin(Request $request) { $this->validate($request, ( 'name' =>'required', 'password' =>'required|min:6' ]); if (Auth::guard('admin')->attempt(['name' => $request->name,'password' => $request->password], $request->get('remember')) ) { return redirect()->intended('/admin'); } return back()->withInput($request->only('name','remember')); } // Add up to the above }
-
Execute the following command to open the controller file that controls login.
$ vi app/Http/Controllers/Auth/RegisterController.php
-
Modify the opened controller file as follows.
<?php namespace App\Http\Controllers\Auth; // Add the following use App\Admin; use Illuminate\Http\Request; // Add the above use App\User; use App\Http\Controllers\Controller; use Illuminate\Support\Facades\Hash; use Illuminate\Support\Facades\Validator; use Illuminate\Foundation\Auth\RegistersUsers; class RegisterController extends Controller { /* |------------------------------------------------- ------------------------- | Register Controller |------------------------------------------------- ------------------------- | | This controller handles the registration of new users as well as their | validation and creation.By default this controller uses a trait to | provide this functionality without requiring any additional code. | */ use RegistersUsers; /** * Where to redirect users after registration. * * @var string */ protected $redirectTo ='/home'; /** * Create a new controller instance. * * @return void */ public function __construct(){ $this->middleware('guest'); //下記を追記する $this->middleware('guest:admin'); } /** * Get a validator for an incoming registration request. * * @param array $data * @return \Illuminate\Contracts\Validation\Validator */ protected function validator(array $data) { return Validator::make($data, [ 'name' => 'required|string|max:255', 'email' => 'required|string|email|max:255|unique:users', 'password' => 'required|string|min:6|confirmed', ]); } /** * Create a new user instance after a valid registration. * * @param array $data * @return \App\User */ protected function create(array $data) { return User::create([ 'name' => $data['name'], 'email' => $data['email'], 'password' => Hash::make($data['password']), ]); } //下記を追記する protected function validatorAdmin(array $data) { return Validator::make($data, [ 'name' => 'required|string|max:255', 'password' => 'required|string|min:6|confirmed', ]); } public function showAdminRegisterForm() { return view('auth.register', ['url' => 'admin']); } protected function createAdmin(Request $request) { $this->validatorAdmin($request->all())->validate(); $admin = Admin::create([ 'name' => $request['name'], 'password' => Hash::make($request['password']), ]); return redirect()->intended('login/admin'); } //上記までを追記する }
-
-
管理者ログインページのビューファイルの修正
-
下記コマンドを実行してログインページのビューファイルを開く。
$ vi resources/views/auth/login.blade.php
-
開いたビューファイルを下記の様に修正する。(修正内容が複雑で分かりにくい時は下記をまるまるコピーして貼り付けてもOKである)
@extends('layouts.app') @section('content') <div class="container"> <div class="row justify-content-center"> <div class="col-md-8"> <div class="card"> <!-- 下記を修正する --> <div class="card-header"> {{ isset($url) ? ucwords($url) : ""}} {{ __('Login') }}</div> <div class="card-body"> @isset($url) <form method="POST" action='{{ url("login/$url") }}' aria-label="{{ __('Login') }}"> @csrf <div class="form-group row"> <label for="name" class="col-sm-4 col-form-label text-md-right">{{ __('Name') }}</label> <div class="col-md-6"> <input id="name" type="name" class="form-control{{ $errors->has('name') ? ' is-invalid' : '' }}" name="name" value="{{ old('name') }}" required autofocus> @if ($errors->has('name')) <span class="invalid-feedback" role="alert"> <strong>{{ $errors->first('name') }}</strong> </span> @endif </div> </div> <div class="form-group row"> <label for="password" class="col-md-4 col-form-label text-md-right">{{ __('Password') }}</label> <div class="col-md-6"> <input id="password" type="password" class="form-control{{ $errors->has('password') ? ' is-invalid' : '' }}" name="password" required> @if ($errors->has('password')) <span class="invalid-feedback" role="alert"> <strong>{{ $errors->first('password') }}</strong> </span> @endif </div> </div> @else <form method="POST" action="{{ route('login') }}" aria-label="{{ __('Login') }}">@csrf <div class="form-group row"> <label for="email" class="col-sm-4 col-form-label text-md-right">{{ __('E-Mail Address') }}</label> <div class="col-md-6"> <input id="email" type="email" class="form-control{{ $errors->has('email') ? ' is-invalid' : '' }}" name="email" value="{{ old('email') }}" required autofocus> @if ($errors->has('email')) <span class="invalid-feedback" role="alert"> <strong>{{ $errors->first('email') }}</strong> </span> @endif </div> </div> <div class="form-group row"> <label for="password" class="col-md-4 col-form-label text-md-right">{{ __('Password') }}</label> <div class="col-md-6"> <input id="password" type="password" class="form-control{{ $errors->has('password') ? ' is-invalid' : '' }}" name="password" required> @if ($errors->has('password')) <span class="invalid-feedback" role="alert"> <strong>{{ $errors->first('password') }}</strong> </span> @endif </div> </div> @endisset <!-- 上記までを修正する --> <div class="form-group row"> <div class="col-md-6 offset-md-4"> <div class="form-check"> <input class="form-check-input" type="checkbox" name="remember" id="remember" {{ old('remember') ? 'checked' : '' }}> <label class="form-check-label" for="remember"> {{ __('Remember Me') }} </label> </div> </div> </div> <div class="form-group row mb-0"> <div class="col-md-8 offset-md-4"> <button type="submit" class="btn btn-primary"> {{ __('Login') }} </button> <a class="btn btn-link" href="{{ route('password.request') }}"> {{ __('Forgot Your Password?') }} </a> </div> </div> </form> </div> </div> </div> </div> </div> @endsection
-
-
管理者登録ページのビューファイルの修正
-
下記コマンドを実行してログインページのビューファイルを開く。
$ vi resources/views/auth/register.blade.php
-
開いたビューファイルを下記の様に修正する。(修正内容が複雑で分かりにくい時は下記をまるまるコピーして貼り付けてもOKである)
@extends('layouts.app') @section('content') <div class="container"> <div class="row justify-content-center"> <div class="col-md-8"> <div class="card"> <!-- 下記を修正する --> <div class="card-header"> {{ isset($url) ? ucwords($url) : ""}} {{ __('Register') }}</div> <div class="card-body"> @isset($url) <form method="POST" action='{{ url("register/$url") }}' aria-label="{{ __('Register') }}"> @csrf <div class="form-group row"> <label for="name" class="col-md-4 col-form-label text-md-right">{{ __('Name') }}</label> <div class="col-md-6"> <input id="name" type="text" class="form-control{{ $errors->has('name') ? ' is-invalid' : '' }}" name="name" value="{{ old('name') }}" required autofocus> @if ($errors->has('name'))<span class="invalid-feedback" role="alert"> <strong>{{ $errors->first('name') }}</strong> </span> @endif </div> </div> <div class="form-group row"> <label for="password" class="col-md-4 col-form-label text-md-right">{{ __('Password') }}</label> <div class="col-md-6"> <input id="password" type="password" class="form-control{{ $errors->has('password') ? ' is-invalid' : '' }}" name="password" required> @if ($errors->has('password')) <span class="invalid-feedback" role="alert"> <strong>{{ $errors->first('password') }}</strong> </span> @endif </div> </div> <div class="form-group row"> <label for="password-confirm" class="col-md-4 col-form-label text-md-right">{{ __('Confirm Password') }}</label> <div class="col-md-6"> <input id="password-confirm" type="password" class="form-control" name="password_confirmation" required> </div> </div> @else <form method="POST" action="{{ route('register') }}" aria-label="{{ __('Register') }}"> @csrf <div class="form-group row"> <label for="name" class="col-md-4 col-form-label text-md-right">{{ __('Name') }}</label> <div class="col-md-6"> <input id="name" type="text" class="form-control{{ $errors->has('name') ? ' is-invalid' : '' }}" name="name" value="{{ old('name') }}" required autofocus> @if ($errors->has('name')) <span class="invalid-feedback" role="alert"> <strong>{{ $errors->first('name') }}</strong> </span> @endif </div> </div> <div class="form-group row"> <label for="email" class="col-md-4 col-form-label text-md-right">{{ __('E-Mail Address') }}</label> <div class="col-md-6"> <input id="email" type="email" class="form-control{{ $errors->has('email') ? ' is-invalid' : '' }}" name="email" value="{{ old('email') }}" required> @if ($errors->has('email')) <span class="invalid-feedback" role="alert"> <strong>{{ $errors->first('email') }}</strong> </span> @endif </div> </div> <div class="form-group row"> <label for="password" class="col-md-4 col-form-label text-md-right">{{ __('Password') }}</label> <div class="col-md-6"> <input id="password" type="password" class="form-control{{ $errors->has('password') ? ' is-invalid' : '' }}" name="password" required> @if ($errors->has('password')) <span class="invalid-feedback" role="alert"> <strong>{{ $errors->first('password') }}</strong> </span> @endif </div> </div> <div class="form-group row"> <label for="password-confirm" class="col-md-4 col-form-label text-md-right">{{ __('Confirm Password') }}</label> <div class="col-md-6"><input id="password-confirm" type="password" class="form-control" name="password_confirmation" required> </div> </div> @endisset <!-- Modify the above --> <div class="form-group row mb-0"> <div class="col-md-6 offset-md-4"> <button type="submit" class="btn btn-primary"> {{ __('Register') }} </button> </div> </div> </form> </div> </div> </div> </div> </div> @endsection
-
-
Creating post-authentication transition page
-
Execute the following command to create a view file.
$ touch resources/views/layouts/auth.blade.php $ touch resources/views/admin.blade.php
-
Execute the following command to open the view file created earlier.
$ vi resources/views/layouts/auth.blade.php
-
Paste the following contents by copy and paste.
<!DOCTYPE html> <html lang="{{ str_replace('_','-', app()->getLocale()) }}"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <!-- CSRF Token --> <meta name="csrf-token" content="{{ csrf_token() }}"> <title>{{config('app.name','Laravel') }}</title> <!-- Scripts --> <script src="{{ asset('js/app.js') }}" defer></script> <!-- Fonts --> <link rel="dns-prefetch" href="https://fonts.gstatic.com"> <link href="https://fonts.googleapis.com/css?family=Raleway:300,400,600" rel="stylesheet" type="text/css"> <!-- Styles --> <link href="{{ asset('css/app.css') }}" rel="stylesheet"> </head> <body> <div id="app"> <nav class="navbar navbar-expand-md navbar-light navbar-laravel"> <div class="container"> <a class="navbar-brand" href="{{ url('/') }}"> {{ config('app.name','Laravel') }} </a> <button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="{{ __('Toggle navigation') }}"> <span class="navbar-toggler-icon"></span> </button> <div class="collapse navbar-collapse" id="navbarSupportedContent"> <!-- Left Side Of Navbar --> <ul class="navbar-nav mr-auto"> </ul> <!-- Right Side Of Navbar --> <ul class="navbar-nav ml-auto"> <!-- Authentication Links --> <li class="nav-item dropdown"> <a id="navbarDropdown" class="nav-link dropdown-toggle" href="#" role="button" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false" v- pre> Hi There <span class="caret"></span> </a> <div class="dropdown-menu dropdown-menu-right" aria-labelledby="navbarDropdown"> <a class="dropdown-item" href="{{ route('logout') }}" onclick="event.preventDefault(); document.getElementById('logout-form').submit();"> {{ __('Logout') }} </a> <form id="logout-form" action="{{ route('logout') }}" method="POST" style="display: none;"> @csrf </form> </div> </li> </ul> </div> </div> </nav> <main class="py-4"> @yield('content') </main> </div> </body> </html>
-
Execute the following command to open the view file created earlier.
$ vi resources/views/admin.blade.php
-
Paste the following contents by copy and paste.```multi_auth_info_limited_laravel_6/resources/views/admin.blade.php @extends(‘layouts.auth’)
@section(‘content’)
Dashboard<div class="card-body"> Hi boss! </div> </div> </div> </div>
Execute the following command to open the view file created earlier.
$ vi resources/views/home.blade.php
Delete the contents already described and paste the following contents by copy and paste.
@extends('layouts.auth') @section('content') <div class="container"> <div class="row justify-content-center"> <div class="col-md-8"> <div class="card"> <div class="card-header">Dashboard</div> <div class="card-body"> Hi there, regular user </div> </div> </div> </div> </div> @endsection
Description of routing and setting of transition page after authentication and description of processing at the time of exception
-
Execute the following command to open the routing file.
$ vi routes/web.php
-
Add as follows.
<?php /* |------------------------------------------------- ------------------------- | Web Routes |------------------------------------------------- ------------------------- | | Here is where you can register web routes for your application. | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('/', function () { return view('welcome'); }); Auth::routes(); Route::get('/home','HomeController@index')->name('home'); // Add the following Route::get('/login/admin','Auth\LoginController@showAdminLoginForm'); Route::get('/register/admin','Auth\RegisterController@showAdminRegisterForm'); Route::post('/login/admin','Auth\LoginController@adminLogin'); Route::post('/register/admin','Auth\RegisterController@createAdmin'); Route::view('/home','home')->middleware('auth'); Route::view('/admin','admin'); // Add the above
Redirect settings
-
Execute the following command to open the middleware file that controls redirection
$ vi app/Http/Middleware/RedirectIfAuthenticated.php
-
Add as follows.
<?php namespace App\Http\Middleware; use Closure; use Illuminate\Support\Facades\Auth; class RedirectIfAuthenticated { public function handle($request, Closure $next, $guard = null) { // add the following if ($guard == "admin" && Auth::guard($guard)->check()) { return redirect('/admin'); } // add up to the above if (Auth::guard($guard)->check()) { return redirect('/home'); } return $next($request); } }
Exception settings
-
Execute the following command to open the handler file.
$ vi app/Exceptions/Handler.php
-
Modify the handler file as shown below.
<?php namespace App\Exceptions; use Exception; use Illuminate\Foundation\Exceptions\Handler as ExceptionHandler; // Add the following use Illuminate\Auth\AuthenticationException; use Auth; // Add the above class Handler extends ExceptionHandler { /** * A list of the exception types that are not reported. * * @var array */ protected $dontReport = [ // ]; /** * A list of the inputs that are never flashed for validation exceptions. * * @var array */ protected $dontFlash = [ 'password', 'password_confirmation', ]; /** * Report or log an exception. * * @param \Exception $exception * @return void */public function report(Exception $exception) { parent::report($exception); } /** * Render an exception into an HTTP response. * * @param \Illuminate\Http\Request $request * @param \Exception $exception * @return \Illuminate\Http\Response */ public function render($request, Exception $exception) { return parent::render($request, $exception); } //下記を追記する protected function unauthenticated($request, AuthenticationException $exception) { if ($request->expectsJson()) { return response()->json(['error' => 'Unauthenticated.'], 401); } if ($request->is('admin') || $request->is('admin/*')) { return redirect()->guest('/login/admin'); } return redirect()->guest(route('login')); } //上記までを追記する }
確認
-
下記コマンドを実行してローカルサーバを起動する。
$ php artisan serve
-
下記にアクセスしてLaravelの初期画面が表示されることを確認する。
-
下記にアクセスし必要情報を入力後「Registar」をクリックする。
-
下記の画面にリダイレクトすることを確認する。一つ前の手順で入力した管理者登録時の情報を入力し「Login」をクリックする。
-
下記のページ「Hi boss!」が表示されれば管理者としてのログインは完了である。
参考文献
-