Creating a site with login function with Laravel with XAMPP (4/4) Search function
Aug 27, 2020
PHP
MySQL
xampp
Laravel
Windows10
Target
**Create a site with user search function using Laravel
. **
Previous article
Create site with login function with Laravel with XAMPP(3/4) User information change function
Search function creation
The search content is a partial match of the user information registered in the table.
Search screen → Create with search result screen
.
Search screen
Create a controller with the following command.
$ php artisan make:controller User/SearchController
With this search function, instead of searching for all partial match values from the search word and then returning the result, the category and column to be searched are specified in advance. Therefore, enter the category to be passed to View on the controller.
<?php
namespace App\Http\Controllers\User;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
class SearchController extends Controller
{
public function search()
{
$category_name = ['ID','NAME','COMMENT'];
return view('user/search',['NAME' => $category_name]);
}
}
On the page to be displayed, create the search function of the category passed from the controller and the content to be searched for in that category.
@extends('layouts.app')
@section('content')
<div class="container">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="card">
<div class="card-header">
User search
</div>
<div class="card-body">
<p>Enter the information of the user you want to search. </p>
<div class="search">
<form method="post" action="result">
<div class="category">
<p>Search item:</p>
<select name="search_category">
<option value = "" selected> Please select</option>
@for($i = 0; $i <3; $i++)
<option value = "{{ $NAME[$i] }}">{{ $NAME[$i] }}</option>
@endfor
</select>
</div>
<div class="word">
<p>Search word:</p>
<input type="text" name="search_word">
</div>
<input type="submit" value="Search">
@csrf
</form>
</div>
<div>
<input type="button" value="Back" onclick="history.back()">
</div>
</div>
</div>
</div>
</div>
</div>
@endsection
The category to be searched can be selected with <select>
, and it is described using @foreach
instead of entering each item individually.
And add the routing process.
Route::get('/home/search','User\SearchController@search');
Don’t forget to add a link to the page you created this time to the transition source home.blade.php
.
<a href="home/user">User search</a>
The following page is completed with the above code.
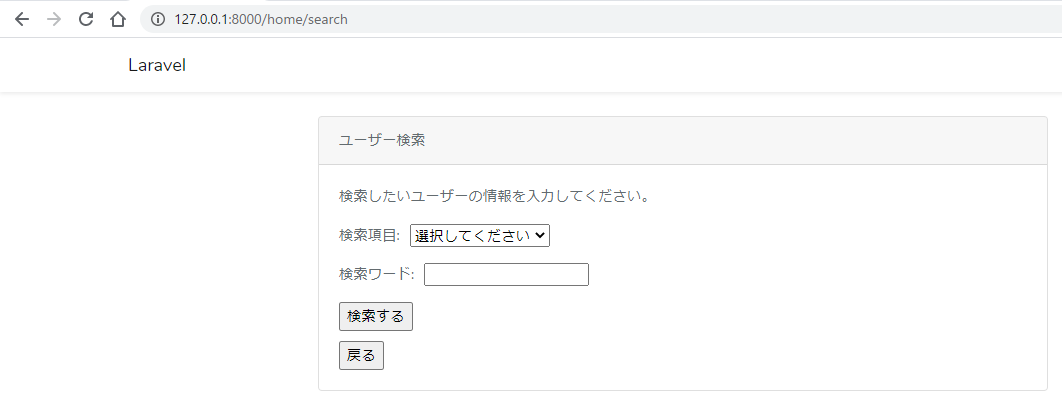
Search result screen
Create the controller with the following command.
$ php artisan make:controller User/ResultController
In this controller, enter the category selected on the search screen
, the word entered on the search screen, and the result of searching the table based on the previous two
.
<?php
namespace App\Http\Controllers\User;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use App\User;
class ResultController extends Controller
{
public function result(Request $request)
{
$category = $request -> input('search_category');
$word = $request -> input('search_word');
$search_data = array(
'category' => $category,
'word' => $word
);
$user_data = User::where($category,'like', "%$word%") -> get();
return view('user/result',['Search' => $search_data,'User' => $user_data]);
}
}
Pass not only the search results, but also the selected category and search word to make it easy to understand what value you searched for.
Partial matching can be searched with where(column name,'like', "%search word%")
.
On the page to display, you just need to display the value passed from the controller.
@extends('layouts.app')
@section('content')
<div class="container">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="card">
<div class="card-header">
search results
</div>
<div class="card-body">
<div class="result">
<form method="post" action="search">
<div class="category">
Search item: {{ $Search['category'] }}
</div>
<div class="word">
Search word: {{ $Search['word'] }}
</div>
@if (isset($User))
<table>
<tr>
<th>ID</th>
<th>NAME</th>
<th>COMMENT</th>
</tr>
@foreach($User as $Data)
<tr>
<td>{{ $Data['id'] }}</td><td>{{ $Data['name'] }}</td>
<td>{{ $Data['comment'] }}</td>
</tr>
@endforeach
</table>
@else
<p>Users matching the search criteria are not registered</p>
@endif
<input type="submit" value="Return to search screen">
@csrf
</form>
</div>
<div class="to_home">
<input type="button" value="To home screen" onclick="/home">
</div>
</div>
</div>
</div>
</div>
</div>
@endsection
Since the search result is not limited to one, I used @foreach
to display all the users who matched by partial match.
The search result screen is a transition with POST
, but enter the routing process when it is accessed with GET
.
Route::get('/home/result','User\SearchController@search');
Route::post('/home/result','User\ResultController@result');
The following page is completed with the above code.
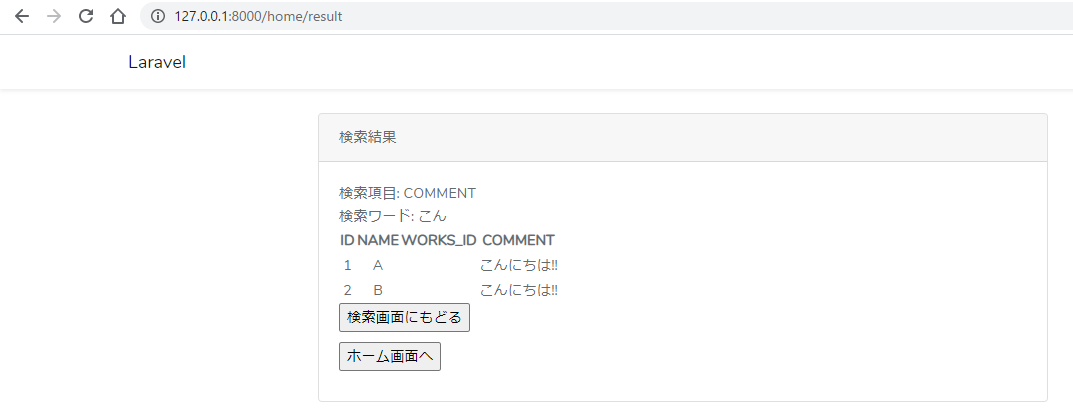
Search result screen → Search screen
Here too, the value on the search result screen is retained so that the screen can be returned to the search screen.
For that, it is necessary to be able to return the value passed in result.blade.php
.
@endif
<input type="hidden" value="{{$Search['category'] }}" name="search_category">
<input type="hidden" value="{{$Search['word'] }}" name="search_word">
<input type="submit" value="Return to search screen">
@csrf
Then, the search screen controller, SearchController.php
, receives the value passed from result.blade.php
and allows it to be passed to the search screen.
public function return_search(Request $request)
{
$category = $request -> input('search_category');
$word = $request -> input('search_word');
$search_data = array(
'category' => $category,
'word' => $word
);
$category_name = ['ID','NAME','COMMENT'];
return view('user/search',['NAME' => $category_name,'Search' => $search_data]);
}
On the search screen, search.blade.php
, it is possible to display the content displayed when transitioning from the search result screen and the previously input value.
// omitted
<form method="post" action="result">
<div class="category">
<p>Search item:</p>
<select name="search_category">
@if(isset($Search))
@for($i = 0; $i <3; $i++)
@if($Search['category'] === $NAME[$i])
<option value = "{{ $NAME[$i] }}" selected>{{ $NAME[$i] }}</option>
@else
<option value = "{{ $NAME[$i] }}">{{ $NAME[$i] }}</option>
@endif
@endfor
@else
<option value = "" selected> Please select</option>
@for($i = 0; $i <3; $i++)
<option value = "{{ $NAME[$i] }}">{{ $NAME[$i] }}</option>
@endfor
@endif
</select>
</div>
<div class="word">
<p>Search word:</p>
@if(isset($Search))
<input type="text" name="search_word" value="{{ $Search['word'] }}">
@else
<input type="text" name="search_word">
@endif
</div>
<input type="submit" value="Search">
@csrf
</form>
@if(isset($Search))
is used to judge whether it is a transition from the search result screen or not depending on whether there is $Search
passed from SearchController.php
.
By adding selected
to the <option>
tag, that value can be selected.
Fill in the routing process and try it out.
Route::post('/home/search','User\SearchController@return_search');
in conclusion
This concludes the study of Laravel
.
Although it is now possible to create basic functions, some functions are not fully used yet, so we will continue learning.